2.3. Communication Examples
2.3.1. Python Example
In this example it is shown how to establish a simple socket communication with the module and request its firmware version (see VER Command).
#!/usr/bin/env python3
import socket
IP = "192.168.0.10"
try:
# Create TCP socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
s.connect((IP, 10001))
# Get VERSION
print("Get Version")
s.sendall("VER:?\r\n".encode())
data = s.recv(2048).decode()
print(repr(data))
# Close socket
s.close()
except :
print("Error: Error in communication with the module")
2.3.2. Putty Example
Putty it is a free telnet client that is available for both Windows and Linux OS. Putty allows sending/receiving commands/replies to/from the power unit.
To establish the communication socket with the unit, it is necessary to configure Putty in the following way:
insert the IP address of the unit,
set the communication port to 10001
set the connection type to Raw.
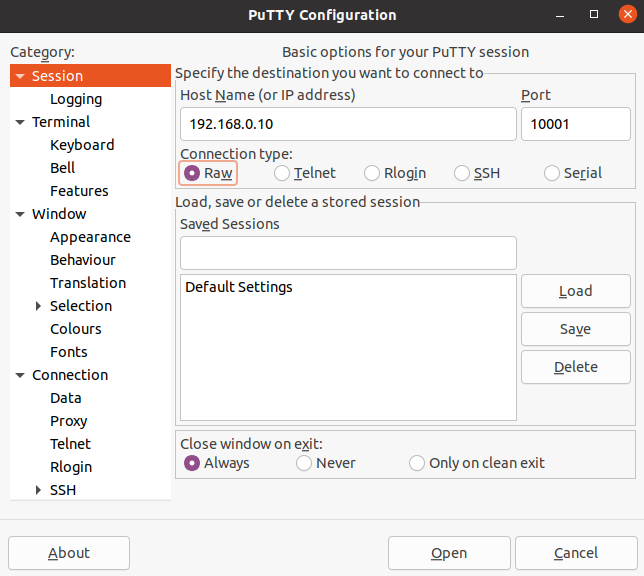
Once the configuration has been completed, it is possible to send the ASCII command directly to the unit:
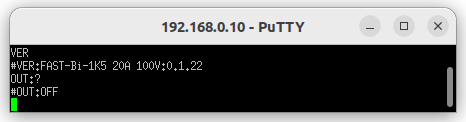